Introduction
Laravel is one of the most popular PHP frameworks, and it is likely to continue to be that way for some time to come. There are many reasons why this is so, like its syntax, robust features, scalability, and, most of all, its high level of security.
Security remains a paramount concern for developers and businesses alike, irrespective of the selection of technology. Laravel, like any other framework, is not immune to security threats.
Conducting an in-depth security audit for a Laravel application involves a comprehensive review of various security aspects to ensure the application is robust and secure.
Security breaches can have devastating consequences for businesses, including data theft, financial loss, and reputation damage. This blog offers a detailed behind-the-scenes look at the process of conducting a Laravel security audit.
What is Laravel Security Audit?
A Laravel security audit systematically examines an application for potential vulnerabilities, misconfigurations, and adherence to security best practices. It ensures that the application aligns with industry standards, such as OWASP Top 10, and safeguards sensitive data. Securing web applications is critical in today's environment of rising cyber threats. Regular security audits are essential to ensure comprehensive protection.
One can assess the application security via a Laravel Security Audit. It helps in evaluating a Laravel application to identify and address vulnerabilities and potential risks that could compromise its security. The audit ensures the application adheres to best practices, protecting sensitive data and preventing cyberattacks.
The main goals of a Laravel Security Audit:
- Identify Laravel Vulnerabilities: Detect security flaws such as SQL injections, XSS attacks, or weak authentication.
- Enhance Security Measures: Reinforce built-in Laravel security features with additional safeguards.
- Ensure Compliance: Meet legal and industry standards (e.g., GDPR, HIPAA, PCI-DSS).
- Maintain User Trust: Protect user data and preserve application integrity.
A Laravel Security Audit is essential for maintaining a secure, compliant, and trustworthy application. It systematically uncovers vulnerabilities and ensures your application is fortified against modern threats. An official Laravel partner will have skilled developers and the necessary resources to deliver top-notch solutions. Acquaint Softtech is one such Laravel development company.
We have over 10 years of experience delivering feature-rich and secure Laravel applications. We are a software development outsourcing company in India with a dedicated team of Laravel developers.
Laravel Security Protocols
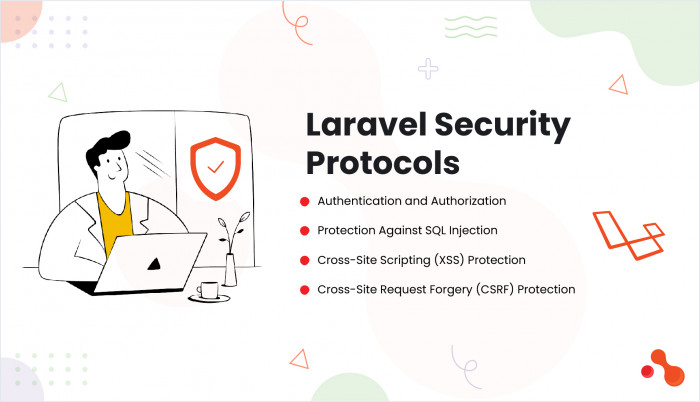
Laravel prioritizes security by incorporating several protocols and best practices to protect web applications from vulnerabilities. This is further verified after the release of version 11, where many more security features were introduced. Below is a comprehensive overview of Laravel's security protocols:
- Authentication and Authorization
- Protection Against SQL Injection
- Cross-Site Scripting (XSS) Protection
- Cross-Site Request Forgery (CSRF) Protection
- Secure File Uploads
- Data Encryption
- Session Security
- HTTPS and HSTS
- API Security
- Error and Exception Handling
- Secure Storage of Environment Variables
- Dependency Management
- Disable Unused Features
- Logging and Monitoring
Common Laravel Security Audit Issues
Laravel is a secure PHP framework. However, it is not uncommon for security vulnerabilities to develop as a result of bad configuration or improper usage of a feature. Some of the common issues found during Laravel security audits include:
- Insufficient input validation
- Missing subresource integrity (SRI)
- Insufficient rate limiting
- Cross-site scripting (XSS)
- Outdated & vulnerable dependencies
- Insecure function use
- Missing security headers
- Missing content security policy (CSP)
- Missing authorization
- Exposed API keys & passwords
Security Audit Process
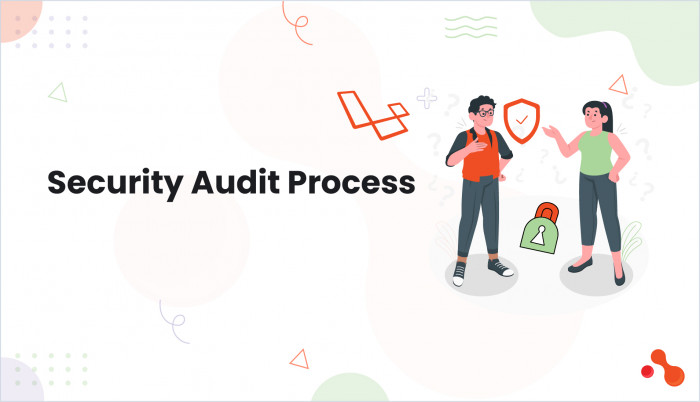
Set up the Scope of the Audit: The first step in a Laravel security audit is defining its scope. This includes:
Identifying Critical Areas:
- Authentication and authorization mechanisms
- Input validation and sanitization
- Application and server configuration
- Data encryption and storage
- API endpoints and integration
Understanding the Application Architecture:
- Documenting the architecture and dependencies
- Mapping data flow within the application
Determining the Audit Type:
- Black-box testing: Testing without access to the source code.
- White-box testing: Testing with full access to the source code.
- Gray-box testing: A combination of both approaches.
Setting Goals:
- Identify and mitigate Laravel vulnerabilities.
- Ensure compliance with legal and regulatory requirements.
- Establish a baseline for ongoing security practices.
Reviewing the Laravel Environment Configuration:
Laravel's .env file is critical for managing environment-specific configurations, but it can also become a security risk if misconfigured.
Checklist for Environment Configuration:
- Ensure the .env file is not accessible publicly. Misconfigured servers can expose this file, revealing sensitive credentials.
- Use strong, randomly generated application keys (APP_KEY) to secure sessions and encrypted data.
- Disable debugging mode (APP_DEBUG=false) in production environments to prevent sensitive information exposure.
Tools Used:
- Manual inspection of configuration files
- Automated tools like Laravel DebugBar for debugging issues
Examining Authentication and Authorization:
Authentication and authorization mechanisms are the backbone of application security. A robust implementation minimizes the risk of unauthorized access.
Authentication:
- Verify the implementation of Laravel’s built-in authentication scaffolding.
- Ensure secure password hashing using bcrypt or Argon2.
- Enforce strong password policies and implement multi-factor authentication (MFA) if possible.
Authorization:
- Review roles and permissions using Laravel policies and gates.
- Check for privilege escalation vulnerabilities.
Session Security:
- Use HTTPS to secure cookies.
- Enable SameSite and HttpOnly attributes for cookies.
- Configure session timeouts and token regeneration.
Tools Used:
- Manual code review
- Laravel’s built-in Auth and Gate facades for validation
Input Validation and Data Sanitization:
Unvalidated or unsanitized user input is a leading cause of security vulnerabilities, including SQL injection, XSS, and command injection.
Best Practices:
- Validation Rules:
- Use Laravel’s request validation methods ($request->validate) to ensure inputs meet specified criteria.
- Avoid custom validation where built-in methods suffice.
- Sanitization:
- Escape output using Laravel’s Blade templating engine ({{ $variable }}) to prevent XSS.
- Use e() for escaping dynamic content in non-Blade contexts.
- SQL Injection Prevention:
- Use Laravel’s query builder or Eloquent ORM to avoid directly executing raw queries.
- Parameterize queries to eliminate injection risks.
- File Upload Security:
- Restrict allowed file types.
- Store uploaded files outside the web root.
- Validate file size and sanitize file names.
- Tools Used:
- OWASP ZAP for input validation testing
- Laravel-specific tools like Larastan for static analysis
Reviewing Encryption and Data Storage Practices:
Sensitive data such as passwords, tokens, and personal information must be encrypted at rest and in transit.
Encryption:
- Ensure sensitive data is encrypted using Laravel’s Crypt facade.
- Use secure algorithms like AES-256 for encryption.
Database Security:
- Use prepared statements and ORM to protect against SQL injection.
- Regularly update database credentials and use role-based access control (RBAC) for database users.
Logs:
- Avoid logging sensitive information in production.
- Rotate logs periodically to prevent unauthorized access.
Tools Used:
- Laravel’s encryption and hashing tools
- Database auditing tools like MySQL Enterprise Audit
Analyzing API Security:
APIs are a common attack vector and require careful attention during a security audit.
Key Areas to Examine:
- Authentication:
- Use token-based authentication (e.g., Laravel Passport or Sanctum).
- Secure API tokens using strong hashing algorithms.
- Rate Limiting:
- Protect against brute force attacks using Laravel’s rate-limiting middleware.
- Input Validation:
- Validate and sanitize all API inputs to prevent injection attacks.
- CORS Policies:
- Restrict origins to trusted domains using Laravel’s CORS middleware.
- Error Handling:
- Ensure error responses do not expose sensitive information.
- Tools Used:
- Postman for manual API testing
- Automated scanners like OWASP ZAP for API vulnerability testing.
Securing Third-Party Dependencies:
Laravel applications often rely on third-party packages and libraries. Unmaintained or insecure dependencies can introduce vulnerabilities.
Best Practices:
- Dependency Management:
- Use Composer to manage dependencies.
- Regularly update dependencies to their latest stable versions.
Review Package Integrity:
- Verify package integrity using checksums.
- Avoid installing unnecessary or unverified packages.
Monitoring Tools:
- Use tools like Snyk or Dependabot to monitor for Laravel vulnerabilities in third-party packages.
Tools Used:
- Composer security audit (composer audit)
- Dependency scanners like Snyk
Conducting Penetration Testing:
Penetration testing simulates real-world attacks to uncover vulnerabilities in the application.
Techniques Used:
- Black-box testing to simulate an attacker without prior knowledge of the system.
- Exploiting common vulnerabilities like XSS, CSRF, and SQL injection.
- Testing for logic flaws and business logic vulnerabilities.
Tools Used:
- OWASP ZAP and Burp Suite for penetration testing
- Kali Linux tools like SQLMap for SQL injection testing
Ensuring Compliance and Reporting:
Security audits must align with relevant compliance standards such as GDPR, HIPAA, or PCI-DSS.
Compliance Checklist:
- Perform data protection impact assessments (DPIAs) where applicable.
- Verify adherence to data retention policies.
- Ensure encryption and logging practices meet regulatory requirements.
Reporting:
- Provide a detailed report outlining identified vulnerabilities, their severity, and recommendations for mitigation.
- Use scoring systems like CVSS to prioritize vulnerabilities.
Tools Used:
- Compliance frameworks like NIST Cybersecurity Framework
- Reporting templates with vulnerability severity matrices.
Implementing Remediation Strategies:
Addressing identified vulnerabilities is as important as finding them. A phased remediation strategy ensures all issues are resolved effectively.
Prioritization:
- Critical vulnerabilities, such as exposed secrets or SQL injection risks, must be addressed immediately.
- Medium and low severity issues can be scheduled for later sprints.
Post-Fix Testing:
- Re-test the application to verify that fixes have resolved the vulnerabilities.
- Conduct regression testing to ensure no new issues have been introduced.
Tools Used:
- Automated testing suites integrated with CI/CD pipelines
- Regression testing tools like PHPUnit for Laravel
Establishing an Ongoing Security Plan:
A one-time security audit is not enough. Continuous monitoring and periodic audits are essential for long-term security.
Ongoing Practices:
- Regularly update Laravel and its dependencies.
- Implement a robust security training program for developers.
- Set up automated alerts for suspicious activities using monitoring tools like Laravel Telescope.
Proactive Measures:
- Perform regular backups and disaster recovery testing.
- Use Web Application Firewalls (WAFs) to block malicious traffic.
Boost Laravel Security
Laravel is the perfect choice for a wide range of applications. However, businesses need to be aware that securing their Laravel application is an ongoing and circular process. It will require the help of an expert team of Laravel developers. Businesses can take advantage of the Laravel development services we offer at Acquaint Softtech.
We are one of the few firms in Asia that is an official Laravel partner. Hence, besides having the necessary resources, you will also hire Laravel developers who always implement the security best practices.
For example, we never take any shortcuts and continuously monitor the security status of the application. Our applications are developed with a high-security principle of the least privileges. We grant sufficient user permission for the application to function but no more than necessary.
Conclusion
A Laravel security audit is an essential step in building secure and reliable web applications. Regular audits, combined with a security-first mindset, empower businesses to stay ahead of evolving threats and maintain user trust in their applications.
Address Laravel vulnerabilities, ensure compliance, and implement current security practices. This is the ideal method to protect sensitive data and safeguard their applications from cyber threats.
However, an in-depth security audit of a Laravel application requires a comprehensive approach. Hire remote developers from a professional firm like Acquaint Softtech to achieve this.
This approach involves authentication, data protection, input validation, session management, and more. We can significantly enhance the security posture of your Laravel application and protect it against common vulnerabilities and threats. Regular audits and updates are essential to maintaining a secure environment as new threats emerge.