Introduction
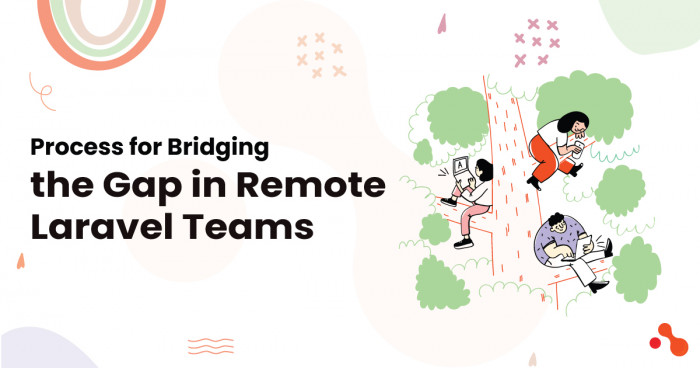
The world of software development has increasingly embraced remote work. Laravel is a robust PHP framework and is becoming one of the leading technologies for remote teams.
There are many advantages of outsourcing Laravel software requirements. This includes access to a global talent pool and flexible work arrangements. But at the same time it also presents unique challenges, particularly around collaboration, communication, and overcoming issues related to code inflexibility.
Bridging the gap in remote Laravel teams and overcoming code inflexibility is critical to fostering collaboration, enhancing productivity, and ensuring the maintainability of a Laravel-based project.
In this article, we will explore how to bridge the gap in remote Laravel teams and tackle the challenge of code inflexibility. We will discuss strategies to enhance communication, collaboration, and productivity while ensuring the code remains flexible, scalable, and maintainable.
Challenges in Remote Laravel Teams
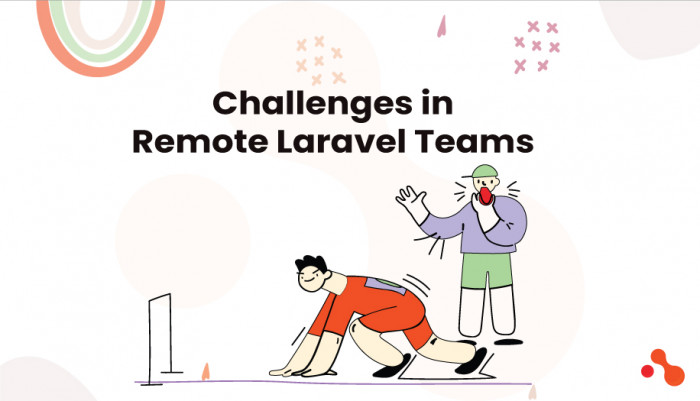
Before diving into solutions, it is essential to understand the key challenges that remote Laravel teams face.
Compared to traditional in-office teams, remote teams often encounter specific issues that can hinder productivity and cohesion. However, this is nothing a professional and well-experienced software development outsourcing company cannot handle.
Acquaint Softtech is one such Laravel development company with the expertise to ensure the delivery of top-notch software. We have over 10 years of experience.
Here are some of the common challenges:
Communication Breakdowns:
One of the biggest challenges for remote teams is communication. Face-to-face interactions are limited, and real-time collaboration becomes more difficult. In a Laravel development environment, this can lead to misunderstandings, missed context, or even mistakes in the codebase, which could snowball into more significant issues.
Time Zone Differences:
Remote teams are often spread across multiple time zones, making it challenging to align schedules for meetings or collaborative work. In Laravel development, this asynchronous communication can lead to delays in code review, deployment, or resolving issues.
Collaborative Code Development:
Working on the same project from different locations can lead to difficulties in managing code consistency, ensuring smooth workflows, and maintaining a high-quality codebase. Laravel teams may struggle with keeping their development practices uniform, leading to code that is difficult to maintain and scale.
Code Inflexibility:
Code inflexibility is a common problem in software development, especially in Laravel projects. Rigid code that is difficult to modify or scale can significantly hamper a remote team's ability to iterate quickly, introduce new features, and adapt to changing requirements.
Difficulty in Building Personal Connections:
Building rapport and personal connections is more difficult when team members don’t interact in person. Remote Laravel teams often focus strictly on work-related discussions, leading to a lack of camaraderie. This can result in lower morale, reduced team cohesion, and a lack of empathy, making it harder to foster a collaborative and supportive team culture.
Managing Cultural Differences:
Remote Laravel teams are often geographically diverse, meaning that team members might come from different cultural backgrounds. Misunderstandings can arise from different communication styles, work ethics, and expectations. This can hinder team alignment and create friction if not properly managed.
Code inflexibility can arise from poor coding practices, lack of adherence to SOLID principles, tight coupling between components, or insufficient use of Laravel’s advanced features like service containers, middleware, and event handling.
Bridging the Communication Gap in Remote Laravel Teams
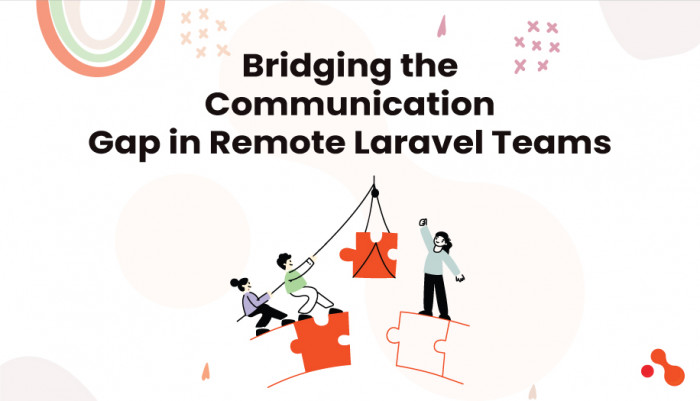
Effective communication is the backbone of any successful remote team. For Laravel developers, communication should be clear, consistent, and supported by appropriate tools and processes to minimize misunderstandings and ensure that everyone stays on the same page.
Utilizing Collaboration Tools:
Leveraging the right collaboration tools can help remote Laravel teams bridge the communication gap. There are several tools specifically designed for remote development teams that allow for better code collaboration, project management, and communication.
Trello and Asana:
These tools allow teams to organize tasks, set deadlines, and monitor project progress in real-time, ensuring that everyone knows what they need to work on.
Jira:
For more complex projects, Jira helps with bug tracking, sprint management, and tracking development stages.
Slack:
Slack provides real-time chat capabilities, channel-based communication, and integrations with other tools. It is one of the most popular communication platforms for remote teams.
Zoom and Microsoft Teams:
For virtual meetings, video calls, and screen sharing, these tools facilitate face-to-face communication, even when working remotely.
GitHub and GitLab:
These platforms allow for code versioning, pull requests, and code reviews, which are crucial for maintaining quality and consistency in the codebase.
Laravel Forge: For deploying and managing Laravel applications, Laravel Forge simplifies remote collaboration by offering a streamlined solution for continuous integration and deployment.
Use Detailed Documentation:
Laravel teams should prioritize documentation for every aspect of their codebase, including the architecture, dependencies, and use cases. This allows developers in different time zones to quickly get up to speed on project requirements.
Scheduled Standups:
Instead of live standup meetings, remote Laravel teams can use asynchronous tools like Slack or Jira to log daily standups. Team members can share their progress, blockers, and plans without requiring everyone to be online simultaneously.
Recorded Demos and Meetings:
Record important demos and meetings so that team members who are unavailable due to time zone differences can watch the recording and stay updated.
Given the nature of remote work, asynchronous communication can often be more effective than trying to align schedules across different time zones. Laravel teams should adopt asynchronous practices for tasks like code review, feedback, and documentation.
Overcoming Code Inflexibility in Laravel
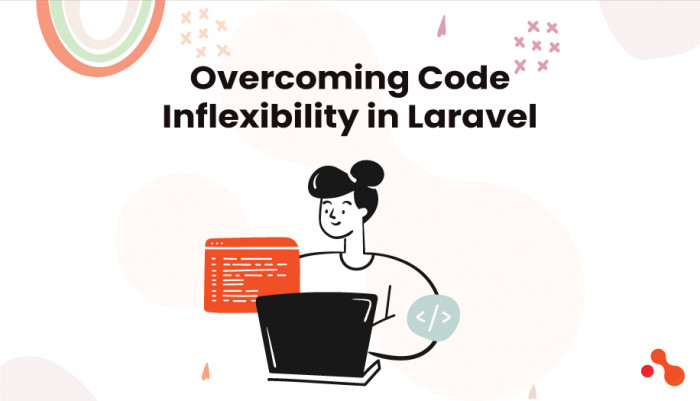
Code inflexibility can be a significant hindrance in Laravel projects, especially when working in a remote environment where collaboration and scalability are key. Writing flexible, maintainable, and scalable code is crucial to keeping the development process smooth and ensuring that the application can adapt to new requirements.
Hire remote developers from Acquaint Softtech to avoid code inflexibility issues. We have already delivered over 5,000 projects worldwide.
Agile Practices:
Implementing Agile methodologies can help remote Laravel teams stay responsive to change. Short sprints, iterative development, and continuous feedback loops allow teams to adapt quickly and efficiently to new requirements or obstacles.
Building Team Cohesion:
Creating a sense of community is vital for remote teams. Virtual coffee chats, social events, and team-building activities foster camaraderie and a supportive environment, crucial for long-term project success.
Adhering to Laravel's Best Practices:
Laravel is a feature-rich framework that provides developers with numerous tools and conventions to create flexible, maintainable code. Adhering to Laravel’s best practices is essential to ensuring the flexibility of your codebase.
Use Laravel’s Service Container:
Laravel’s service container is a powerful tool for dependency injection. It allows you to bind interfaces to implementations, enabling developers to write loosely coupled, flexible code. By relying on contracts (interfaces) instead of concrete implementations, you can easily swap implementations without affecting the entire codebase.
php
Copy code
interface PaymentGateway {
public function charge($amount);
}
class StripeGateway implements PaymentGateway {
public function charge($amount) {
// Charge via Stripe
}
}
class PayPalGateway implements PaymentGateway {
public function charge($amount) {
// Charge via PayPal
}
}
app()->bind(PaymentGateway::class, StripeGateway::class);
Use Eloquent ORM Properly:
While Eloquent ORM is a powerful tool for working with databases, improper use of Eloquent can lead to code inflexibility, especially when the relationships between models are not well-structured. Follow best practices for creating relationships between models and using query scopes to keep the codebase clean and maintainable.
Keep Controllers Slim:
Laravel promotes the MVC architecture, but controllers can become bloated if too much logic is placed inside them. Instead, make use of service classes, form requests, and observers to extract business logic from the controller layer, making the code more maintainable.
Follow SOLID Principles:
Following SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) principles helps ensure that your code is flexible and maintainable. In Laravel, this can be achieved by keeping classes focused on a single responsibility, using polymorphism to extend functionality, and relying on dependency injection.
Using Laravel's Modular Features:
Laravel offers various modular features that allow for better organization and scalability of the codebase. Using these features ensures that different components of the application remain decoupled and can evolve independently.
Middleware:
Middleware is a powerful feature in Laravel that allows you to filter HTTP requests before they reach your controller. By placing common logic like authentication, logging, or throttling in middleware, you keep your controllers slim and your application logic modular.
Service Providers:
Laravel’s service providers are essential for bootstrapping application services. If your application has different service layers or external dependencies, organizing them in service providers ensures they can be easily modified or replaced without affecting the entire application.
Implementing Continuous Integration (CI) and Continuous Deployment (CD):
Automating testing, deployment, and code quality checks can significantly improve the flexibility of your code and reduce the risk of issues arising from human error. Implementing Continuous Integration (CI) and Continuous Deployment (CD) ensures that code changes are regularly tested, making it easier to catch bugs and maintain high-quality code in a remote team setting.
GitHub Actions:
GitHub Actions allows for automation of workflows like testing, building, and deploying code directly from your GitHub repository.
Laravel Envoyer:
Envoyer is a zero-downtime deployment service built for Laravel applications. It allows you to seamlessly deploy your Laravel app without downtime, making remote team collaboration more efficient.
Version Control and Code Review Practices:
One of the primary methods for overcoming code inflexibility and ensuring a consistent codebase in remote teams is to enforce strict version control and code review practices. Version control systems like Git, when used effectively, allow multiple developers to work on the same codebase without causing conflicts.
Best Practices for Git:
Encourage developers to work on separate feature branches instead of committing directly to the main branch. This helps isolate changes and prevents conflicts.
Code Reviews:
Implement a strict code review process where senior team members or peers review the code for consistency, adherence to best practices, and potential issues.
Merge Strategies:
Use strategies like "squash and merge" or "rebase and merge" to keep the Git history clean and concise.
Automated Testing:
Automated testing is crucial for ensuring that code remains flexible and maintainable. By writing unit tests, integration tests, and end-to-end tests for your Laravel application, you can easily refactor code without fear of breaking existing functionality.
Testing Tools for Laravel:
- PHPUnit: Laravel uses PHPUnit, which allows you to write and run unit tests to verify that individual components of your application are functioning as expected. Furthermore, Laravel 11 ships with PEST, although it continues to support PHPUnit.
- Laravel Dusk: Dusk provides browser automation testing for Laravel applications. It is beneficial for testing the UI/UX of your application.
- Refactoring and Code Reviews: Code refactoring should be a regular part of the development process to ensure that the code remains flexible, scalable, and easy to modify. Regular code reviews and refactoring sessions help remove technical debt and improve the overall quality of the codebase.
Encourage remote Laravel teams to dedicate time to refactoring and improving parts of the code that are inflexible or difficult to work with. Use automated tools like PHPStan and Laravel Shift to identify code smells and areas that need improvement.
Fostering Collaboration in Remote Laravel Teams
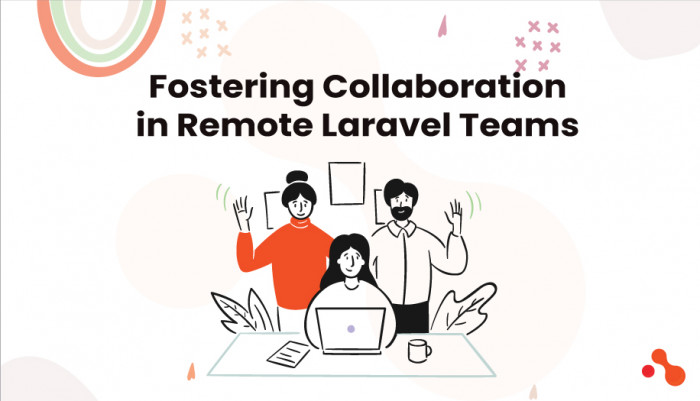
Fostering collaboration is essential to the success of remote Laravel teams. Teams need to be empowered with the right tools, practices, and mindset to work together effectively, regardless of their physical location.
When you hire Lavavel developers to build your robust solution, it is vital that you ensure the firm has the ability to foster collaboration as well. Here are some of the standard techniques for collaboration with remote laravel teams:
Establish Coding Standards:
Coding standards help maintain consistency across the codebase, making it easier for remote teams to collaborate. Laravel teams should agree on specific coding standards, such as PSR-12, and use tools like PHP CodeSniffer to enforce them.
Conduct Regular Code Reviews:
Regular code reviews help catch potential issues early and ensure that the code remains clean, flexible, and scalable. Use GitHub or GitLab pull requests for code review and encourage developers to provide constructive feedback to one another.
Businesses can benefit from the outsourcing services offered by the experts at Acquaint Softtech. We also provide IT staff augmentation services for those looking for more flexibility.
Conclusion
Bridging the gap in remote Laravel teams and overcoming code inflexibility are two major challenges faced by modern software development teams. However, with the right strategies, tools, and practices, these challenges can be effectively addressed.
By focusing on communication, collaboration, and adherence to Laravel’s best practices, remote teams can create flexible, scalable, and maintainable codebases that thrive in the dynamic environment of modern web development.
From leveraging communication tools and adopting asynchronous work practices to implementing modular architecture and continuous integration, Laravel teams can overcome the hurdles of remote collaboration and create successful, robust applications.
With a focus on fostering a culture of collaboration, innovation, and technical excellence, remote Laravel teams can overcome code inflexibility and stay ahead in today’s fast-paced software development landscape.