Introduction
Laravel is a well-known PHP framework. Some of the factors that make it popular include its elegant syntax, documentation, community support, and powerful features. It also happens to be one of the most secure frameworks. However, like any web framework, Laravel applications are susceptible to various security vulnerabilities if not properly managed.
Security concerns in web applications can pose serious risks to both the application's integrity and the privacy of its users. They arise from a combination of human error, misconfiguration, and the increasing complexity of modern web applications. Security failures and successes in real-world Laravel applications can provide valuable insights into how security practices, or the lack thereof, can impact web applications.
In web development, security is a paramount concern that can determine an application's success or failure. This article helps us understand the common Laravel security pitfalls and successes in real-world applications.
Security Concerns in Laravel
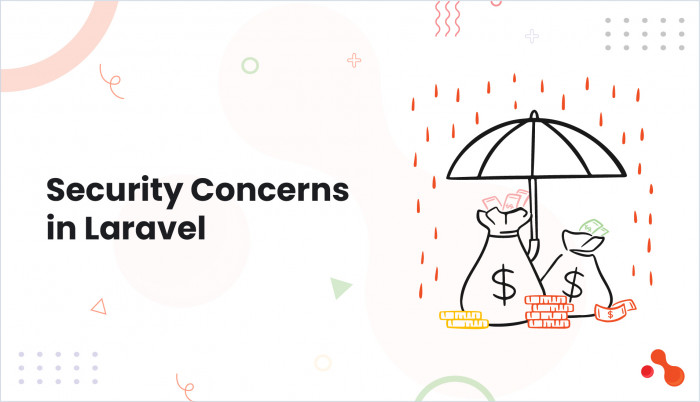
Laravel is one of the most popular web development frameworks. It provides a wide range of built-in security features. However, developers often overlook certain key security aspects, leading to vulnerabilities.
The following are some of the most common security concerns in Laravel apps:
SQL Injection:
SQL Injection (SQLi) is one of the most prevalent security threats in web applications. It occurs when a malicious user manipulates input fields to inject SQL queries into your database, which can potentially result in data breaches, data loss, or even total control over the application. While Laravel's Eloquent ORM and query builder protect against SQL injection by automatically escaping parameters, developers need to be mindful when writing raw queries or manipulating user input.
Cross-Site Scripting (XSS):
Cross-Site Scripting (XSS) attacks occur when malicious scripts are injected into a website and executed in the user’s browser. This can result in stolen cookies, session hijacking, and manipulation of user accounts. Laravel offers built-in mechanisms to protect against XSS attacks, but developers must ensure they are properly utilizing them.
Cross-Site Request Forgery (CSRF):
Cross-Site Request Forgery (CSRF) attacks occur when an attacker tricks a user into submitting unwanted actions, like changing account details or making a purchase, without their knowledge. Laravel provides a CSRF protection mechanism by default, ensuring that all forms include a CSRF token to validate the authenticity of requests.
Authentication and Authorization Vulnerabilities:
Authentication and authorization are critical aspects of Laravel security, ensuring that only legitimate users can access certain parts of the application. Laravel offers authentication mechanisms that are out-of-the-box, but improper implementation can lead to unauthorized access and privilege escalation attacks.
Session Hijacking and Session Fixation:
Session hijacking occurs when an attacker steals a user’s session ID to gain unauthorized access to their account. Session fixation attacks, on the other hand, force a user’s session ID to be set to a specific value, allowing an attacker to impersonate the user.
File Upload Vulnerabilities:
Handling file uploads can expose your application to various security risks, including arbitrary file uploads and execution of malicious code. Laravel provides mechanisms for securely uploading and validating files, but developers must follow best practices to avoid security risks.
Insecure Dependencies:
Modern applications rely on various third-party packages and libraries. Using outdated or vulnerable dependencies can expose your application to security risks. Laravel projects often include multiple packages from the Composer repository, and it is essential to ensure that these dependencies are secure.
Some of the other security concerns in Laravel include insecure direct object reference (IDOR), bad security configurations, and API issues.
Case Studies
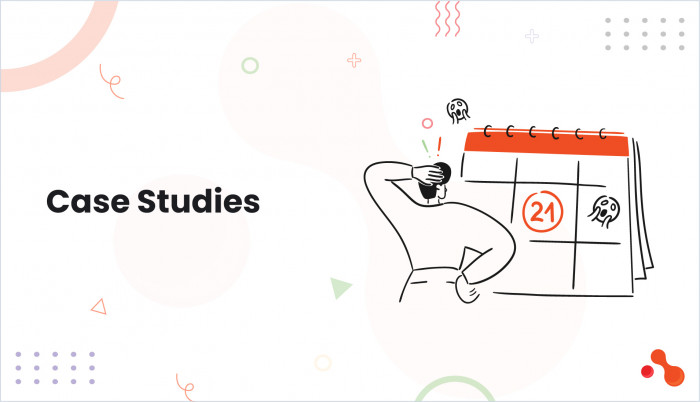
Businesses need to be aware of the benefits of developing secure Laravel solutions. These case studies do just that by showcasing how, by incorporating security measures, they were able to be successful:
Multi-Factor Authentication (MFA) Implementation : Case 1
- Success Overview: MFA has been increasingly adopted by Laravel applications to secure user authentication and reduce the risks of account compromise.
- Implementation: One eCommerce platform built on Laravel successfully integrated MFA into its authentication process. This step added an extra layer of protection beyond just passwords.
- Impact: Drastically reduced account takeovers, even when some users had weak passwords. Boosts user trust in the security of the platform.
- Lessons Learned: Implement MFA in applications handling sensitive data or financial transactions. Laravel has native support for integrating MFA through packages like Laravel Fortify, simplifying implementation.
Encryption of Sensitive Data : Case 2
- Success Overview: One healthcare company using Laravel for its patient management system successfully secured sensitive patient data using encryption for both data at rest and in transit.
- Implementation: The company utilized Laravel’s built-in encryption methods to encrypt sensitive data such as patient health records, payment details, and other PII (Personally Identifiable Information). They also enforced HTTPS for all communications.
- Impact: Even in the event of data theft or unauthorized access, the encrypted data was unusable by attackers. The company passed strict healthcare compliance audits (e.g., HIPAA).
- Lessons Learned: Always encrypt sensitive data, especially when dealing with PII, financial information, or healthcare data. Use Laravel’s native encryption features or third-party libraries to ensure the integrity of encrypted data.
Regular Security Audits and Patching : Case 3
- Success Overview: A major SaaS (Software as a Service) company running on Laravel implemented a rigorous schedule of security audits, vulnerability scanning, and patching.
- Implementation: The company conducted quarterly security audits and employed automated vulnerability scanners to catch potential issues in both custom code and third-party packages. They also maintained a strict patching schedule to address newly discovered vulnerabilities.
- Impact: The application remained free from major security breaches for over five years.
- The SaaS company built a strong reputation for security, attracting larger enterprise clients.
- Lessons Learned: Regularly audit application code and dependencies for vulnerabilities. Implement automated tools for vulnerability scanning and keep both Laravel and third-party libraries up to date.
API Rate Limiting and Throttling : Case 4
- Success Overview: An online platform with a public API built on Laravel successfully implemented Rate limiting and throttling to prevent abuse and DDoS (Distributed Denial of Service) attacks.
- Implementation: Using Laravel’s built-in API rate limiting features, the platform was able to mitigate brute-force attacks and prevent abuse from automated scripts attempting to overwhelm the system.
- Impact: Prevented DDoS attacks that could have taken down the platform and improved the platform's resilience and availability, maintaining service even during attempted attacks.
- Lessons Learned: Implement Rate limiting for all public APIs to prevent abuse. Laravel's throttle middleware is easy to configure and should be applied to sensitive routes like login and API endpoints.
Common Laravel Security Pitfalls
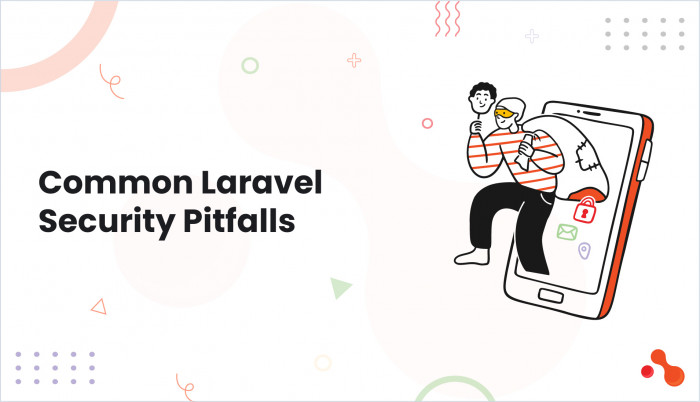
Security audits of Laravel applications have revealed a trend of common issues that developers face. One of the most prevalent problems is insufficient input validation, which can lead to injection attacks and other vulnerabilities.
Laravel provides a $fillable property on models to guard against mass-assignment vulnerabilities, but developers must use it judiciously to prevent unintended access control issues.
Another often overlooked security measure is Subresource Integrity (SRI). SRI helps protect against compromised third-party scripts by verifying an integrity hash before loading the resource. Its underuse leaves many applications vulnerable to malicious code injections.
Rate limiting is another critical security feature that is frequently missing in Laravel applications. Proper Rate limiting can prevent bot attacks and abuse, especially in sensitive areas like authentication and user account queries.
Best Practices To Follow
Hire remote developers with extensive experience in developing top-notch solutions. Ones that incorporate a high level of security and have exceptional skills. They should ideally be following best practices for security as well.
Acquaint Softtech is one such software development outsourcing company with over 10 years of experience. We have a dedicated team of Laravel developers along with a very state-of-the-art QA team to deliver flawless solutions. Our developers have access to all the necessary resources as well, especially since we are one of the few firms that is an official Laravel Partner.
To ensure the security of Laravel applications, when you hire Laravel developers, ensure they adhere to the following best practices:
- Validate Input Rigorously: Use the validation features to check all incoming data thoroughly.
- Employ SRI: Implement Subresource Integrity for all third-party scripts and styles.
- Implement Rate Limiting: Use Laravel's Rate-limiting features to protect against brute force attacks.
- Stay Updated: Keep Laravel and all dependencies up to date to benefit from the latest security patches.
- Use Trusted Packages: Only use packages from trusted sources and maintain them regularly.
- Conduct Regular Audits: Perform security audits and penetration testing to identify and address vulnerabilities.
- Configuration is Critical: Many Laravel security failures are a result of misconfigurations (e.g., exposing .env files, improper CSRF protection). Always audit configurations and review deployment settings to minimize exposure.
- Update and Patch Regularly: Keeping Laravel, third-party libraries, and dependencies up to date is crucial to prevent exploitation of known vulnerabilities.
Would you like assistance in adopting any of these practices for your Laravel projects? Do not hesitate to get in touch with Acquaint Softtech. We offer outsourcing as well as IT staff augmentation services.
Conclusion
Laravel stands out as a framework that prioritizes security, but it requires developers to be vigilant and proactive. By understanding common security failures and implementing best practices, a Laravel development company applications that are not only functional but also secure.
Adopt Laravel security best practices such as input validation, encryption, and secure authentication. Regularly update code and dependencies.Conduct thorough security audits and implement monitoring to detect potential vulnerabilities early.
Take advantage of the Laravel development services provided by an official Laravel partner like Acquaint Softtech and gain the upper edge.