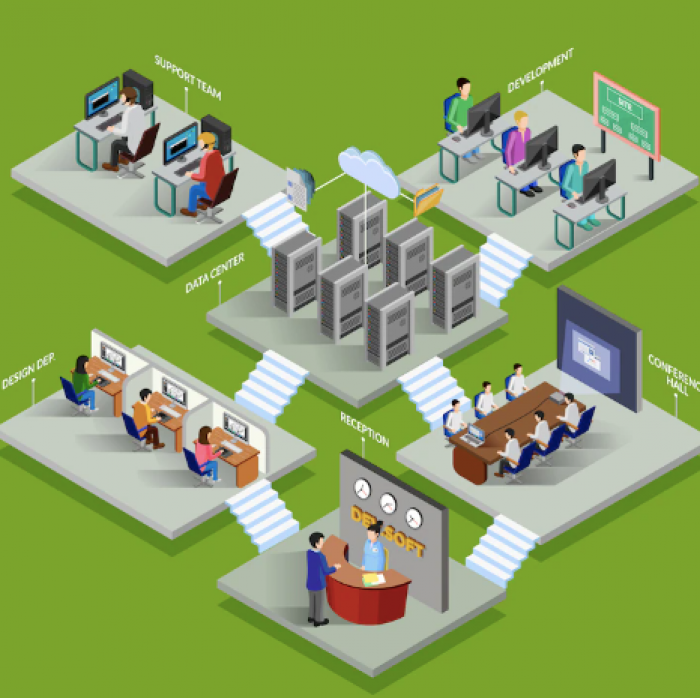
In Spring Boot, layered architecture is crucial in designing and organizing applications.
Layers provide a modular and structured approach to application development, enabling better separation of concerns and improved maintainability.
Spring Boot interview questions are among the most common questions asked in technical interviews.
In this article, we will delve into the various layers of Spring Boot, exploring their purpose, responsibilities, and interactions.
Layers in Spring Boot
Presentation Layer
The presentation and web layers handle the user interface and interaction in a Spring Boot application.
It acts as a bridge between the user and the underlying application logic. This layer includes components such as controllers, which receive and process user requests, and view templates, which generate the user interface.
The presentation layer renders data, handles form submissions, and manages user sessions. It plays a crucial role in defining the overall user experience and orchestrating the flow of information between the user interface and other application layers.
Controller Layer
The controller layer is a crucial component in the layered architecture of Spring Boot applications. It acts as an intermediary between the presentation and service layers, responsible for handling user requests and orchestrating the application's business logic.
In the controller layer, incoming requests are received, validated, and processed. It maps the request data to appropriate service methods and coordinates the data flow between the user interface and the underlying layers.
The controller layer plays a pivotal role in ensuring the separation of concerns and properly handling user interactions, making it a vital part of Spring Boot applications.
Service Layer
The service layer in Spring Boot plays a crucial role in implementing the application's business logic and coordinating the flow of data between the controller and the repository layers.
It acts as an intermediary between the presentation and data access layers, encapsulating complex operations and business rules.
The service layer provides a cohesive set of services that handle tasks such as data validation, transformation, transaction management, and orchestration of multiple repository operations.
By separating the business logic into the service layer, developers can achieve modularity, reusability, and easier testing, promoting clean and maintainable code.
Repository Layer
The repository layer in Spring Boot acts as an interface between the service layer and the underlying data storage system, such as a database.
It abstracts the data access operations and provides a standardized way to interact with the data. The repository layer is responsible for querying, inserting, updating, and deleting data from the data storage system.
It encapsulates the data access logic and provides a higher-level abstraction for the service layer, promoting modularity and separation of concerns.
Spring Boot's repository layer is often implemented using Spring Data, simplifying data access by providing ready-to-use CRUD operations and query methods based on conventions and annotations.
Data Access Layer
The Data Access Layer in Spring Boot handles the interaction between the application and the underlying data storage system, such as a database.
It abstracts the specific details of the data access implementation, allowing the upper layers to work with a consistent and unified interface.
The Data Access Layer typically utilizes repositories or DAOs (Data Access Objects) to encapsulate the logic for querying, inserting, updating, and deleting data.
Spring Boot provides powerful data access features, such as the Spring Data framework, simplifying data access through its repository abstractions and automatic query generation capabilities.
This layer plays a crucial role in enabling efficient and organized data access operations within Spring Boot applications.
Persistence Layer
The persistence layer in Spring Boot is responsible for handling the storage and retrieval of data. It is an interface between the application and the underlying data storage system, such as a relational database or NoSQL datastore.
The persistence layer involves saving, updating, retrieving, and deleting data. In Spring Boot, the persistence layer is often implemented using frameworks like Spring Data JPA, Hibernate, or MyBatis. Frameworks-related spring boot interview questions are also commonly asked during technical interviews.
These frameworks provide abstractions and APIs that simplify database interactions, reduce boilerplate code, and offer features like object-relational mapping (ORM) and transaction management. The persistence layer is crucial in ensuring data integrity and persistence within the application.
Business Logic Layer
The Business Logic Layer in a software application contains the core functionality and rules that drive the business processes.
It encapsulates the logic and algorithms that manipulate data, enforces business rules, and implement specific operations.
The Business Logic Layer ensures that the application adheres to the business requirements and provides a centralized and reusable set of services.
Separating the business logic from other layers, such as the presentation or data access layer, promotes modularity, maintainability, and code reusability.
Dependency Injection Layer
The dependency injection (DI) layer in Spring Boot plays a crucial role in managing the dependencies between application components.
DI enables loose coupling and promotes modularity by decoupling the creation and configuration of objects from their usage.
This layer facilitates the injection of dependencies into various components, such as controllers, services, and repositories, allowing them to collaborate seamlessly.
Spring Boot's DI mechanism simplifies the configuration and management of dependencies, making the application more flexible, maintainable, and testable.
Configuration Layer
The configuration layer in Spring Boot handles the configuration of various application components and external resources.
It provides a centralized mechanism for managing properties, setting up connection details, defining beans, and configuring other application-specific settings.
The configuration layer often utilizes annotations, XML, or external configuration files to define and manage the application's behaviour.
Testing Layer
The testing layer focuses on ensuring the correctness and reliability of the application.
It involves writing and executing various tests, including unit, integration, and end-to-end tests.
The testing layer verifies that each component within the different layers functions as expected and satisfies the specified requirements.
Integration Layer
The integration layer handles the integration of external systems, services, or APIs into the application. It enables communication and interoperability between the application and external entities.
The integration layer may involve handling API calls, integrating with third-party services, or implementing message queues for asynchronous processing.
All the above layers-related spring boot interview questions are asked in the technical interviews.
Pro Tip: SQL and Spring Boot are distinct technologies that often work together to build robust and scalable applications. Therefore, thoroughly practicing SQL interview questions while preparing spring boot interview questions are mandatory.
Conclusion
Understanding the layers in Spring Boot is vital for building robust and scalable applications. Each layer has a specific responsibility and contributes to the overall architecture and functionality of the application.
By adopting a layered approach, developers can achieve separation of concerns, modularity, and maintainability. Spring Boot's framework and ecosystem provide the necessary tools and abstractions to facilitate the implementation and interaction of these layers.
By leveraging the power of Spring Boot's layered architecture, developers can design and develop applications that are flexible, extensible, and easier to manage and test.